负载均衡算法是用于在多个服务器之间分配请求的技术,目的是优化资源使用、最大化吞吐量、最小化响应时间,并避免任何单一资源过载,以下是几种常见的负载均衡算法及其Java代码实现:
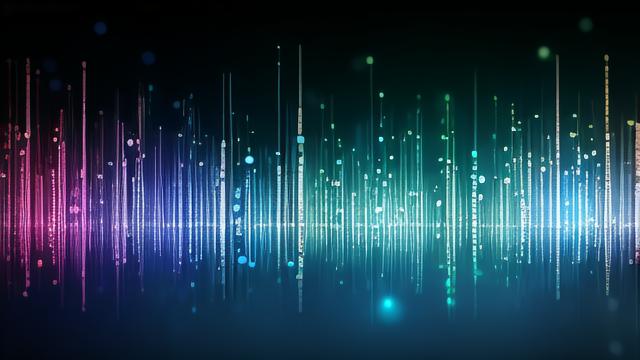
1、轮询法(Round Robin)
概念:按顺序将每个新连接的请求分配给下一个服务器,最终将所有请求平分给所有服务器。
优点:绝对公平。
缺点:无法根据服务器性能分配请求,无法合理利用服务器资源。
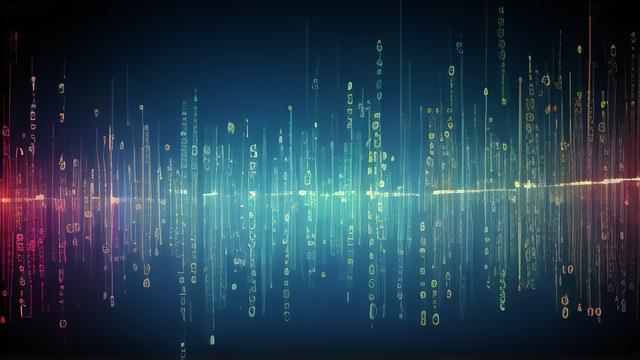
Java代码实现:
import java.util.*; import java.util.concurrent.ConcurrentHashMap; public class TestRoundRobin { static Map<String, Integer> ipMap = new HashMap<>(); static { ipMap.put("192.168.13.1", 1); ipMap.put("192.168.13.2", 1); ipMap.put("192.168.13.3", 1); } Integer pos = 0; public String RoundRobin() { Map<String, Integer> ipServerMap = new ConcurrentHashMap<>(); ipServerMap.putAll(ipMap); Set<String> ipSet = ipServerMap.keySet(); ArrayList<String> ipList = new ArrayList<>(ipSet); synchronized (pos) { if (pos >= ipSet.size()) { pos = 0; } return ipList.get(pos++); } } public static void main(String[] args) { TestRoundRobin testRoundRobin = new TestRoundRobin(); for (int i = 0; i < 10; i++) { System.out.println(testRoundRobin.RoundRobin()); } } }
2、加权轮询法(Weighted Round Robin)
概念:每个服务器根据其权重接受相应比例的连接请求,如果第三台机器的处理能力是第一台的两倍,那么它将接受两倍的连接数量。
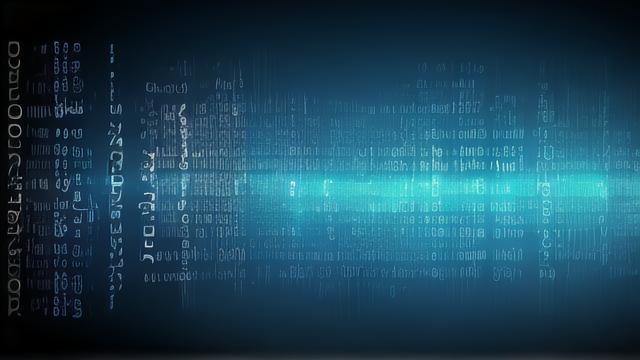
优点:可以根据服务器性能合理分配请求。
缺点:可能导致某些服务器长时间执行耗时大的请求,压力较大。
Java代码实现:
import java.util.*; import java.util.concurrent.ConcurrentHashMap; public class TestWeightRobin { static Map<String, Integer> ipMap = new HashMap<>(); static { ipMap.put("192.168.13.1", 1); ipMap.put("192.168.13.2", 2); ipMap.put("192.168.13.3", 4); } Integer pos = 0; Integer totalWeight = 0; Integer currentIndex = 0; public String WeightRobin() { Map<String, Integer> ipServerMap = new ConcurrentHashMap<>(); ipServerMap.putAll(ipMap); totalWeight = ipServerMap.values().stream().mapToInt(Integer::intValue).sum(); Iterator<String> ipIterator = ipServerMap.keySet().iterator(); while (ipIterator.hasNext()) { String serverName = ipIterator.next(); Integer weight = ipServerMap.get(serverName); for (int i = 0; i < weight; i++) { currentIndex = (currentIndex + 1) % totalWeight; if (currentIndex == 0) { return serverName; } } } return null; // Should never reach here } public static void main(String[] args) { TestWeightRobin testWeightRobin = new TestWeightRobin(); for (int i = 0; i < 10; i++) { System.out.println(testWeightRobin.WeightRobin()); } } }
3、随机法(Random)
概念:通过系统随机函数,根据后台服务器列表的大小值来随机选取一台服务器进行访问。
优点:简单易实现。
缺点:有效性受到质疑,因为随机性可能导致负载不均。
Java代码实现:
import java.util.*; import java.util.concurrent.ConcurrentHashMap; public class TestRandom { static Map<String, Integer> ipMap = new HashMap<>(); static { ipMap.put("192.168.13.1", 1); ipMap.put("192.168.13.2", 2); ipMap.put("192.168.13.3", 4); } public String Random() { Map<String, Integer> ipServerMap = new ConcurrentHashMap<>(); ipServerMap.putAll(ipMap); Set<String> ipSet = ipServerMap.keySet(); ArrayList<String> ipList = new ArrayList<>(ipSet); Random random = new Random(); int randomPos = random.nextInt(ipList.size()); return ipList.get(randomPos); } public static void main(String[] args) { TestRandom testRandom = new TestRandom(); for (int i = 0; i < 10; i++) { System.out.println(testRandom.Random()); } } }
4、加权随机法(Weighted Random)
概念:根据服务器的权重进行随机选择,权重越大,被选中的概率越高。
优点:可以更合理地分配请求。
缺点:当权重设置过大时,可能会导致List过大。
Java代码实现:
import java.util.*; import java.util.concurrent.ConcurrentHashMap; public class TestWeightRandom { static Map<String, Integer> ipMap = new HashMap<>(); static { ipMap.put("192.168.13.1", 1); ipMap.put("192.168.13.2", 2); ipMap.put("192.168.13.3", 4); } public String WeightRandom() { Map<String, Integer> ipServerMap = new ConcurrentHashMap<>(); ipServerMap.putAll(ipMap); Set<String> ipSet = ipServerMap.keySet(); ArrayList<String> ipList = new ArrayList<>(ipSet); Random random = new Random(); int totalWeight = ipServerMap.values().stream().mapToInt(Integer::intValue).sum(); int randomPos = random.nextInt(totalWeight); for (String server : ipList) { randomPos -= ipServerMap.get(server); if (randomPos <= 0) { return server; } } return null; // Should never reach here } public static void main(String[] args) { TestWeightRandom testWeightRandom = new TestWeightRandom(); for (int i = 0; i < 10; i++) { System.out.println(testWeightRandom.WeightRandom()); } } }
5、源地址哈希法(IP_Hash)
概念:通过对客户端IP地址进行哈希计算,得到一个数值,用该数值对服务器列表取模,从而确定访问哪台服务器。
优点:同一个IP地址的客户端会被分配到同一台后端服务器,适用于需要会话保持的场景。
缺点:如果服务器列表变化,哈希结果也会变化,可能导致会话失效。
Java代码实现:
import java.util.*; import java.util.concurrent.ConcurrentHashMap; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.math.BigInteger; public class IpHash { static Map<String, Integer> ipMap = new HashMap<>(); static { ipMap.put("192.168.13.1", 1); ipMap.put("192.168.13.2", 2); ipMap.put("192.168.13.3", 4); } private static int getHash(String ip) throws NoSuchAlgorithmException { MessageDigest md5 = MessageDigest.getInstance("MD5"); md5.update(ip.getBytes()); byte[] digest = md5.digest(); BigInteger no = new BigInteger(1, digest); return no.intValue() & Integer.MAX_VALUE; // Ensure positive hash value } public String IpHash() throws NoSuchAlgorithmException { Map<String, Integer> ipServerMap = new ConcurrentHashMap<>(); ipServerMap.putAll(ipMap); Set<String> ipSet = ipServerMap.keySet(); ArrayList<String> ipList = new ArrayList<>(ipSet); String clientIp = "192.168.13.1"; // Example client IP address int hash = getHash(clientIp); return ipList.get(hash % ipList.size()); } public static void main(String[] args) throws NoSuchAlgorithmException { IpHash ipHash = new IpHash(); for (int i = 0; i < 10; i++) { System.out.println(ipHash.IpHash()); } } }
相关问题与解答栏目:
问题1: 为什么加权轮询法在某些情况下会导致负载不均?
回答: 加权轮询法在处理耗时较长的请求时,可能会导致某些服务器长时间处于高负载状态,因为它们的权重较高,会接收更多的请求,这会导致负载不均衡,特别是在有大量耗时请求的情况下,为了解决这个问题,可以使用平滑加权轮询算法,动态调整服务器的权重,以平衡负载。
以上就是关于“负载均衡算法代码”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!